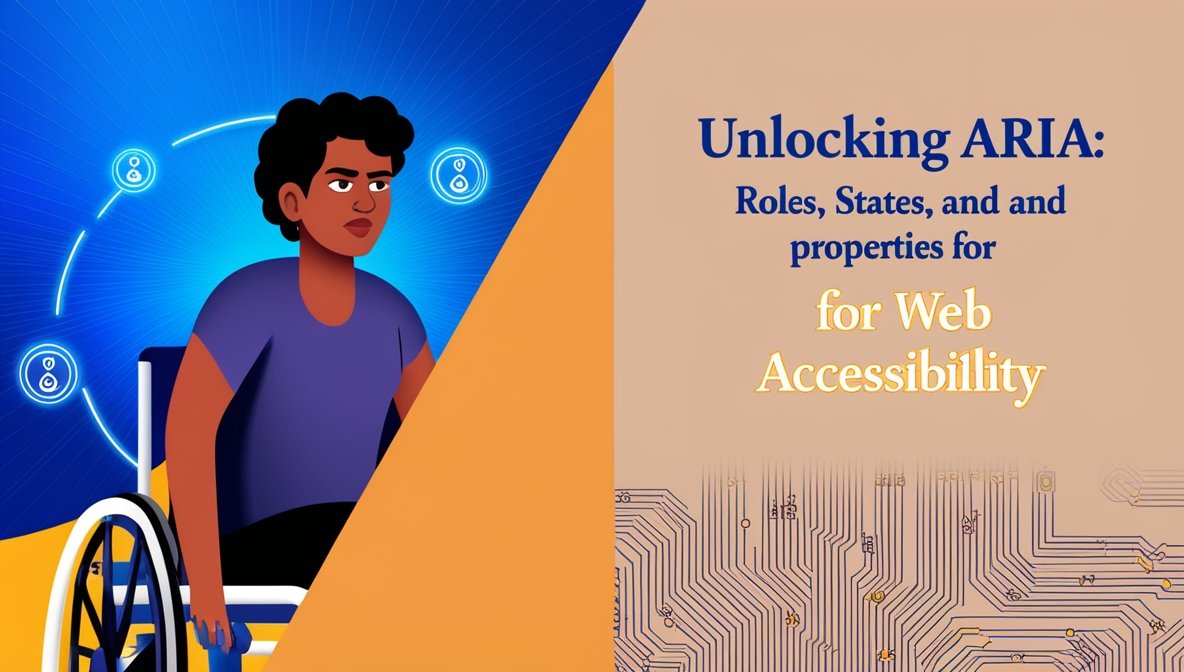
Web accessibility is a critical aspect of modern web design, ensuring that all users, regardless of their abilities, can access and navigate web content effectively. ARIA (Accessible Rich Internet Applications) is a set of attributes that improve the accessibility of dynamic content and user interface elements, especially when traditional HTML alone cannot provide sufficient information for assistive technologies. In this article, we’ll explore ARIA, focusing on its key components: Roles, States, and Properties, with code examples to help you understand their application.
What is ARIA?
ARIA stands for Accessible Rich Internet Applications. It is a set of attributes that can be added to HTML elements to enhance the accessibility of web content, especially when building dynamic or complex user interfaces. ARIA helps convey important information to users of assistive technologies (like screen readers) that may not be available through standard HTML tags.
ARIA is particularly valuable when dealing with JavaScript-driven interactions such as custom widgets, modal dialogs, accordions, and more. By using ARIA roles, states, and properties, we can ensure that these dynamic elements are accessible to all users.
Key Components of ARIA
1. ARIA Roles
ARIA roles define the type of element on the page, providing essential information about its behavior and purpose. Roles help assistive technologies interpret and communicate the function of an element.
Common ARIA roles include:
- button: Represents a clickable button.
- navigation: Denotes a navigation section.
- dialog: Represents a modal or dialog window.
- menu: Indicates a menu or list of options.
- tablist: Denotes a list of tabs.
- tabpanel: Represents the content associated with a tab.
2. ARIA States
ARIA states describe the dynamic conditions of an element that can change over time. These states provide important feedback to users, especially when the element’s state is altered via user interaction.
Common ARIA states include:
- aria-expanded: Indicates whether a collapsible element (like an accordion) is expanded or collapsed.
- aria-checked: Indicates whether a checkbox or radio button is selected.
- aria-disabled: Shows whether an element is disabled.
- aria-selected: Represents whether an item (such as a tab or option) is selected.
3. ARIA Properties
ARIA properties provide additional information about elements, giving context to how they interact with other elements on the page.
Common ARIA properties include:
- aria-controls: Specifies the element(s) controlled by the current element.
- aria-labelledby: Provides a label for an element, often used in conjunction with
role="dialog"
orrole="alert"
. - aria-hidden: Tells assistive technologies whether an element is visible or hidden.
ARIA Example: Accordion with Roles, States, and Properties
To better understand how ARIA roles, states, and properties work together, let’s look at a practical example: a simple accordion. An accordion is a UI component that expands and collapses sections when clicked.
Code Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>ARIA Accordion Example</title>
<style>
.accordion-header {
cursor: pointer;
padding: 10px;
border: 1px solid #ccc;
background-color: #f9f9f9;
}
.accordion-content {
border: 1px solid #ccc;
border-top: none;
padding: 10px;
display: none;
}
.accordion-content[aria-hidden="false"] {
display: block;
}
</style>
</head>
<body>
<div class="accordion">
<!-- Accordion Item 1 -->
<div>
<button
id="accordion-header-1"
class="accordion-header"
role="button"
aria-expanded="false"
aria-controls="accordion-content-1"
>
Section 1
</button>
<div
id="accordion-content-1"
class="accordion-content"
role="region"
aria-labelledby="accordion-header-1"
aria-hidden="true"
>
<p>This is the content for Section 1.</p>
</div>
</div>
<!-- Accordion Item 2 -->
<div>
<button
id="accordion-header-2"
class="accordion-header"
role="button"
aria-expanded="false"
aria-controls="accordion-content-2"
>
Section 2
</button>
<div
id="accordion-content-2"
class="accordion-content"
role="region"
aria-labelledby="accordion-header-2"
aria-hidden="true"
>
<p>This is the content for Section 2.</p>
</div>
</div>
</div>
<script>
// JavaScript to toggle accordion sections
const headers = document.querySelectorAll('.accordion-header');
headers.forEach(header => {
header.addEventListener('click', () => {
const expanded = header.getAttribute('aria-expanded') === 'true';
const content = document.getElementById(header.getAttribute('aria-controls'));
// Toggle the state
header.setAttribute('aria-expanded', !expanded);
content.setAttribute('aria-hidden', expanded);
});
});
</script>
</body>
</html>
Explanation of ARIA Roles, States, and Properties in the Example:
Roles:
role="button"
: Applied to the accordion header to specify that the header is interactive, functioning as a button.role="region"
: Used on the accordion content to signify that the section is a distinct content area. It also helps assistive technologies identify regions of the page that can be navigated.
States:
aria-expanded="false"
: Indicates that the accordion section is collapsed initially. When clicked, this state is toggled totrue
(expanded).aria-hidden="true"
: Used on the content to indicate that it is initially hidden. It is toggled tofalse
when expanded, meaning it is visible.
Properties:
aria-controls="accordion-content-1"
: Specifies that the button controls the content of the section withid="accordion-content-1"
.aria-labelledby="accordion-header-1"
: Associates the accordion content with the button, so that assistive technologies can tell which header labels the content.
Why ARIA is Important
ARIA plays a crucial role in making interactive and dynamic content accessible, especially when native HTML elements don’t provide sufficient information. For example, an accordion widget built entirely with <div>
elements might not be recognized as a collapsible section without ARIA attributes.
By using roles, states, and properties, we ensure that assistive technologies such as screen readers can understand and communicate the purpose of elements. This makes our web applications more inclusive for users with disabilities, leading to a better and more accessible user experience.
Conclusion
ARIA (Accessible Rich Internet Applications) attributes, including roles, states, and properties, provide essential accessibility information for complex, dynamic web elements. They allow us to create interactive components like accordions, modals, and custom form controls that are fully usable by people with disabilities, ensuring compliance with web accessibility standards and making the web a more inclusive place.
By applying these ARIA features, developers can improve their websites’ usability, ensuring that everyone, regardless of ability, has equal access to digital content. Always remember to test your accessible features with screen readers and other assistive technologies to ensure they provide the best possible experience for all users.